WinFormsでも、以下の組み合わせで疑似的にEnter/Leaveのタイミングを検出することが出来ます。
取得したマウスポインタ位置を元にRectangle.Contains メソッドで範囲チェックを行う。
参考記事:スクリーン座標←→クライアント座標の変換を行うには?
ある座標や領域がコントロールの領域内に含まれるているかを確認するには?
使用するデータは以下のいずれか。
以前の状態がウィンドウの内か外であったかを記憶しておき、変化があったらEnter/Leaveのタイミング発生と判断する。
LowLevelMouseProcを使ったプログラムソースはこちら(タイマーの例は割愛)
namespace WindowsFormsApp1
{
using System;
using System.Windows.Forms;
using System.Runtime.InteropServices;
public partial class Form1 : Form
{
public delegate int HookProc(int nCode, IntPtr wParam, IntPtr lParam);
HookProc MouseHookLLProcedure;
static int hLLHook = 0;
static bool bPrevMouseIn = false;
public const int HC_ACTION = 0;
public const int WH_MOUSE_LL = 14;
[StructLayout(LayoutKind.Sequential)]
public class POINT
{
public int x;
public int y;
}
[StructLayout(LayoutKind.Sequential)]
public class MouseLLHookStruct
{
public POINT pt;
public int mouseData;
public int flags;
public int time;
public int dwExtraInfo;
}
[DllImport("kernel32.dll", CharSet = CharSet.Auto, CallingConvention = CallingConvention.StdCall)]
public static extern IntPtr GetModuleHandle(string lpModuleName);
[DllImport("user32.dll", CharSet = CharSet.Auto, CallingConvention = CallingConvention.StdCall)]
public static extern int SetWindowsHookEx(int idHook, HookProc lpfn, IntPtr hInstance, int threadId);
[DllImport("user32.dll", CharSet = CharSet.Auto, CallingConvention = CallingConvention.StdCall)]
public static extern bool UnhookWindowsHookEx(int idHook);
[DllImport("user32.dll", CharSet = CharSet.Auto, CallingConvention = CallingConvention.StdCall)]
public static extern int CallNextHookEx(int idHook, int nCode, IntPtr wParam, IntPtr lParam);
public Form1()
{
InitializeComponent();
bPrevMouseIn = false;
}
private void Form1_Activated(object sender, EventArgs e)
{
if (hLLHook == 0)
{
MouseHookLLProcedure = new HookProc(Form1.MouseLLHookProc);
hLLHook = SetWindowsHookEx(WH_MOUSE_LL, MouseHookLLProcedure, GetModuleHandle(null), 0);
}
}
private static int MouseLLHookProc(int nCode, IntPtr wParam, IntPtr lParam)
{
if (nCode == HC_ACTION)
{
MouseLLHookStruct MyMouseHookStruct = (MouseLLHookStruct)Marshal.PtrToStructure(lParam, typeof(MouseLLHookStruct));
Form1 tempForm = Form.ActiveForm as Form1;
if (tempForm != null)
{
bool fInMouse = false;
// 内側領域の場合
fInMouse = tempForm.RectangleToScreen(tempForm.ClientRectangle).Contains(MyMouseHookStruct.pt.x, MyMouseHookStruct.pt.y);
// 外側領域の場合
//fInMouse = tempForm.DesktopBounds.Contains(MyMouseHookStruct.pt.x, MyMouseHookStruct.pt.y);
if (fInMouse != bPrevMouseIn)
{ // EnterまたはLeaveの発生
tempForm.textBox1.AppendText("X= " + MyMouseHookStruct.pt.x.ToString("d4") +
" Y= " + MyMouseHookStruct.pt.y.ToString("d4") + (fInMouse ? " Enter" : " Leave") + "\r\n");
bPrevMouseIn = fInMouse; // 今回情報の記憶
}
}
}
return CallNextHookEx(hLLHook, nCode, wParam, lParam);
}
private void Form1_FormClosed(object sender, FormClosedEventArgs e)
{
if (hLLHook != 0)
{
bool ret = UnhookWindowsHookEx(hLLHook);
hLLHook = 0;
}
}
}
}
フォームのソースはこちら
namespace WindowsFormsApp1
{
partial class Form1
{
private System.ComponentModel.IContainer components = null;
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows フォーム デザイナーで生成されたコード
private void InitializeComponent()
{
this.button1 = new System.Windows.Forms.Button();
this.button2 = new System.Windows.Forms.Button();
this.button3 = new System.Windows.Forms.Button();
this.textBox1 = new System.Windows.Forms.TextBox();
this.SuspendLayout();
// button1
this.button1.Location = new System.Drawing.Point(-1, -1);
this.button1.Name = "button1";
this.button1.Size = new System.Drawing.Size(128, 96);
this.button1.TabIndex = 0;
this.button1.Text = "button1";
this.button1.UseVisualStyleBackColor = true;
// button2
this.button2.Location = new System.Drawing.Point(146, -1);
this.button2.Name = "button2";
this.button2.Size = new System.Drawing.Size(128, 96);
this.button2.TabIndex = 1;
this.button2.Text = "button2";
this.button2.UseVisualStyleBackColor = true;
// button3
this.button3.Location = new System.Drawing.Point(294, -1);
this.button3.Name = "button3";
this.button3.Size = new System.Drawing.Size(128, 96);
this.button3.TabIndex = 2;
this.button3.Text = "button3";
this.button3.UseVisualStyleBackColor = true;
// textBox1
this.textBox1.Location = new System.Drawing.Point(0, 192);
this.textBox1.Multiline = true;
this.textBox1.Name = "textBox1";
this.textBox1.ScrollBars = System.Windows.Forms.ScrollBars.Vertical;
this.textBox1.Size = new System.Drawing.Size(421, 206);
this.textBox1.TabIndex = 3;
// Form1
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 12F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(421, 397);
this.Controls.Add(this.textBox1);
this.Controls.Add(this.button3);
this.Controls.Add(this.button2);
this.Controls.Add(this.button1);
this.Name = "Form1";
this.Text = "Form1";
this.Activated += new System.EventHandler(this.Form1_Activated);
this.FormClosed += new System.Windows.Forms.FormClosedEventHandler(this.Form1_FormClosed);
this.ResumeLayout(false);
this.PerformLayout();
}
#endregion
private System.Windows.Forms.Button button1;
private System.Windows.Forms.Button button2;
private System.Windows.Forms.Button button3;
private System.Windows.Forms.TextBox textBox1;
}
}
表示の例がこちら
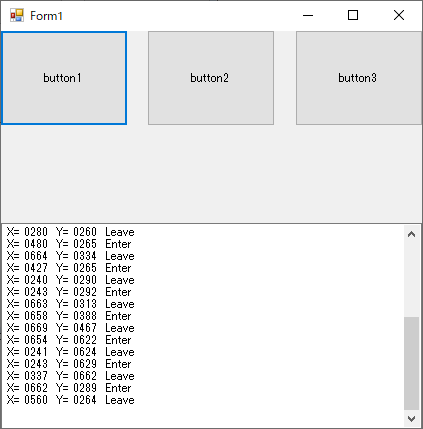
追記:
@Uncle-Kei さんの指摘はもっともだと思いますが、それはまた別の課題ということで、それに関連する記事を探してみました。
Windowsが行っているであろう処理を二重に行うことになるでしょうが、WindowFromPoint function を応用することで自分のウィンドウが最上位かどうかの判断が出来るのではないでしょうか?
指定されたポイントを含むウィンドウへのハンドルを取得します。
戻り値は、ポイントを含むウィンドウへのハンドルです。指定されたポイントにウィンドウが存在しない場合、戻り値はNULLです。ポイントが静的テキストコントロール上にある場合、戻り値は静的テキストコントロール下のウィンドウへのハンドルです。
WindowFromPointの関数は、ポイントがウィンドウ内であっても、非表示または無効になっウィンドウへのハンドルを取得しません。アプリケーションは、制限のない検索のためにChildWindowFromPoint関数を使用する必要があります。
以下この辺の記事で情報を取得する処理を扱っており、それが自分のプロセスのものであると判断できれば対応する処理を行うとか出来そうです。
VB/Delphi/C++等でそのまま使うのは出来ませんが参考にはなるでしょう。
指定したウィンドウの情報を取得
ホーム >プログラム >Delphi 6 ローテクTips >マウスカーソル下のウィンドウを取得
Windows APIメモ WindowFromPoint
第102章 ウィンドウタイトルを変更する
他にはこんな記事も
How to get the z-order in windows?
Given a hwnd, determine if that window is not hidden by other windows (Z-Ordering)
いずれにしても、元々の処理と併せてさらに重い処理になるでしょうけど。